Consider you're working on a project that involves creating music with artificial intelligence. Can ChatGPT make music. Everything is going smoothly until you realize you need to add sound to your program, and time is of the essence. This is where Python Playsound comes in.
Python Playsound is a Python library that plays sound files with just a single function call. It supports WAV and MP3 files and is compatible with Windows, Mac, and Linux operating systems.
This guide will supply you with all the information you need to get started with Python Playsound so you can get on with your project. Musicfy's solution, Python Playsound, lets you quickly play sound files without any complex setup.
If you can't wait to use Musicfy's Free AI Voice Generator, you can try out 1000+ celebrity voices, like:
You can use all of these voices and 1000+ more for free today on create.musicfy.lol!
Table Of Contents
Complete Step-by-Step Guide On How To Use Musicfy's AI Voice Generator
Create Viral Music In Seconds For Free with Musicfy's AI Music Generator
What is Python Playsound?
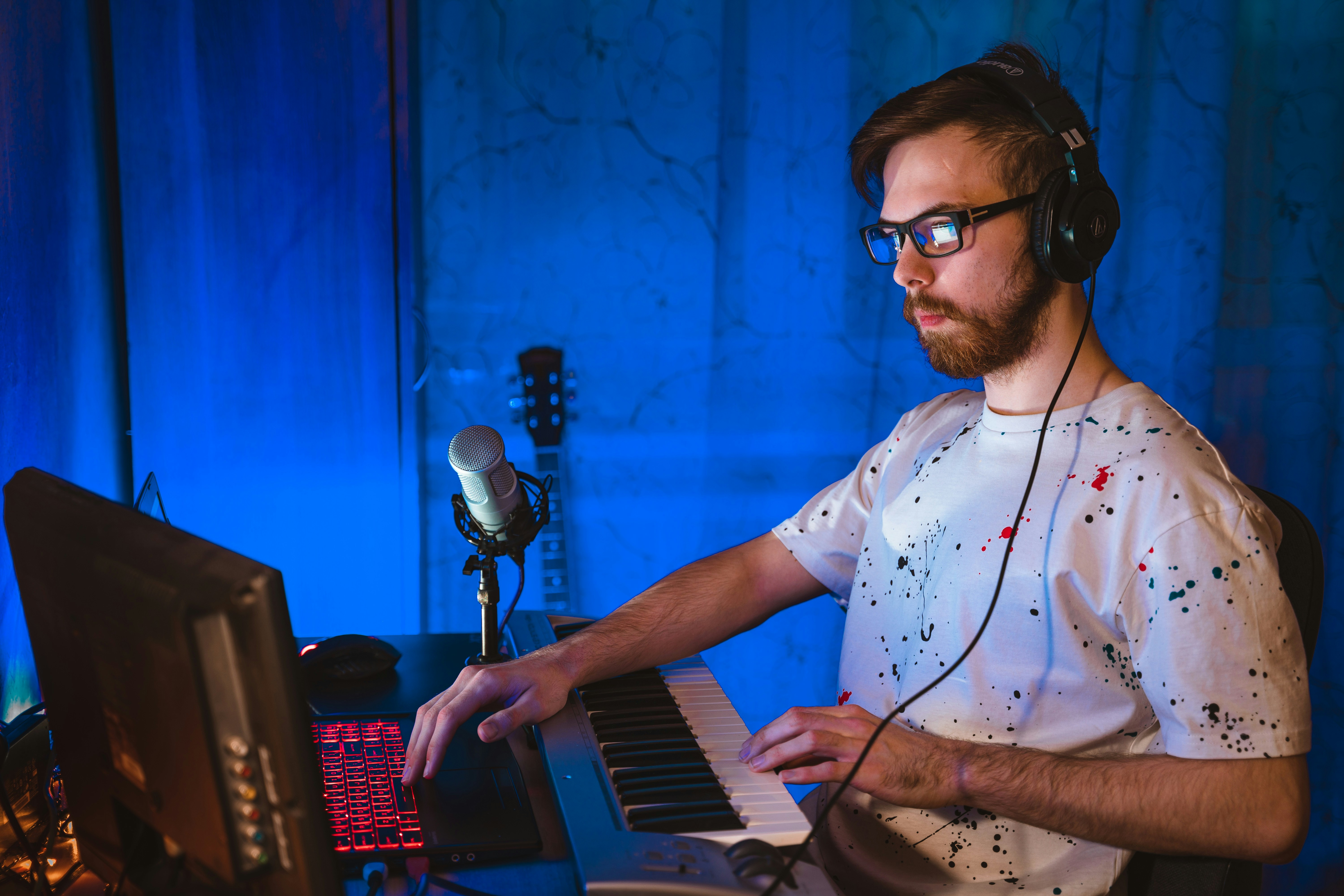
Python Playsound is a lightweight and user-friendly Python module for playing sound files. It provides a simple way to incorporate audio into your projects, requiring minimal setup and coding knowledge. Playsound is especially useful for developers who need quick, straightforward audio playback without advanced manipulation features.
Key Features of Python Playsound
Cross-Platform Compatibility
Playsound works smoothly on Windows, macOS, and Linux. While it functions well across platforms, there may be slight variations in performance depending on the operating system.
Ease of Use
Playsound is incredibly simple, requiring just one line of code to play a sound file. This simplicity makes it ideal for beginners and those looking for rapid development.
Support for Popular Formats
It primarily supports .mp3 and .wav files, making it versatile for most basic audio needs. These formats are widely used and easily accessible.
Minimal Dependencies
Playsound doesn’t require additional software or frameworks to function, unlike other audio libraries. This keeps the installation process straightforward and reduces setup time.
Why Use Python Playsound?
Playsound is a go-to solution for developers who want to integrate sound into their Python projects without investing time in learning advanced audio libraries. Here’s why it stands out:
Simplicity
You don’t need extensive knowledge of sound programming to use Playsound. Its syntax is intuitive and minimalistic, allowing you to focus on your project instead of troubleshooting complex code.
Speed and Efficiency
Playsound is lightweight and fast, making it suitable for real-time applications or simple notifications.
Versatility Across Applications
Playsound can be used in various projects, including games, educational apps, automation scripts, and productivity tools.
No Overhead
It doesn’t bog down your system with unnecessary processing or memory usage, making it ideal for lightweight applications.
Applications of Python Playsound
Here’s how Python Playsound can be applied in real-world scenarios:
Gaming
Add sound effects like button clicks, level-up notifications, or victory sounds. Example: A simple sound that plays when a player completes a level.
Educational Tools
Use Playsound in educational apps to play pronunciation guides, quizzes, or auditory instructions. Example: A language learning app that plays a word’s pronunciation when clicked.
Automation Scripts
Sound alerts will be used to notify users when tasks are completed. Example: A script that plays a chime when data processing is finished.
Productivity Applications
Provide audio feedback for completed actions, such as task completion or reminders. Example: A to-do list app that plays a congratulatory tone when a task is checked off.
Related Reading
• ChatGPT for Music
• AI Music Prompt
• ChatGPT Music Composition
• • How Is AI Music Made
Complete Step-by-Step Guide On How To Use Musicfy's AI Voice Generator
Just a reminder, if you can't wait and are eager to use Musicfy's Free Spongebob AI Voice Generator, you can try out our free Spongebob Squarepants AI voice generator, and 100+ more celebrity voices and popular voices on create.musicfy.lol for free right now!
Video Guide
Written Guide
1. Download An Audio File or Find A Youtube Link For A Song That You Want To Use for A Voice Over or An AI Song Cover
2. Go To Create.musicfy.lol
You will land on this page

3. Upload Your Audio File
You can upload the audio file, or you can upload a Youtube link
Upload Audio File:

Upload Youtube Link:

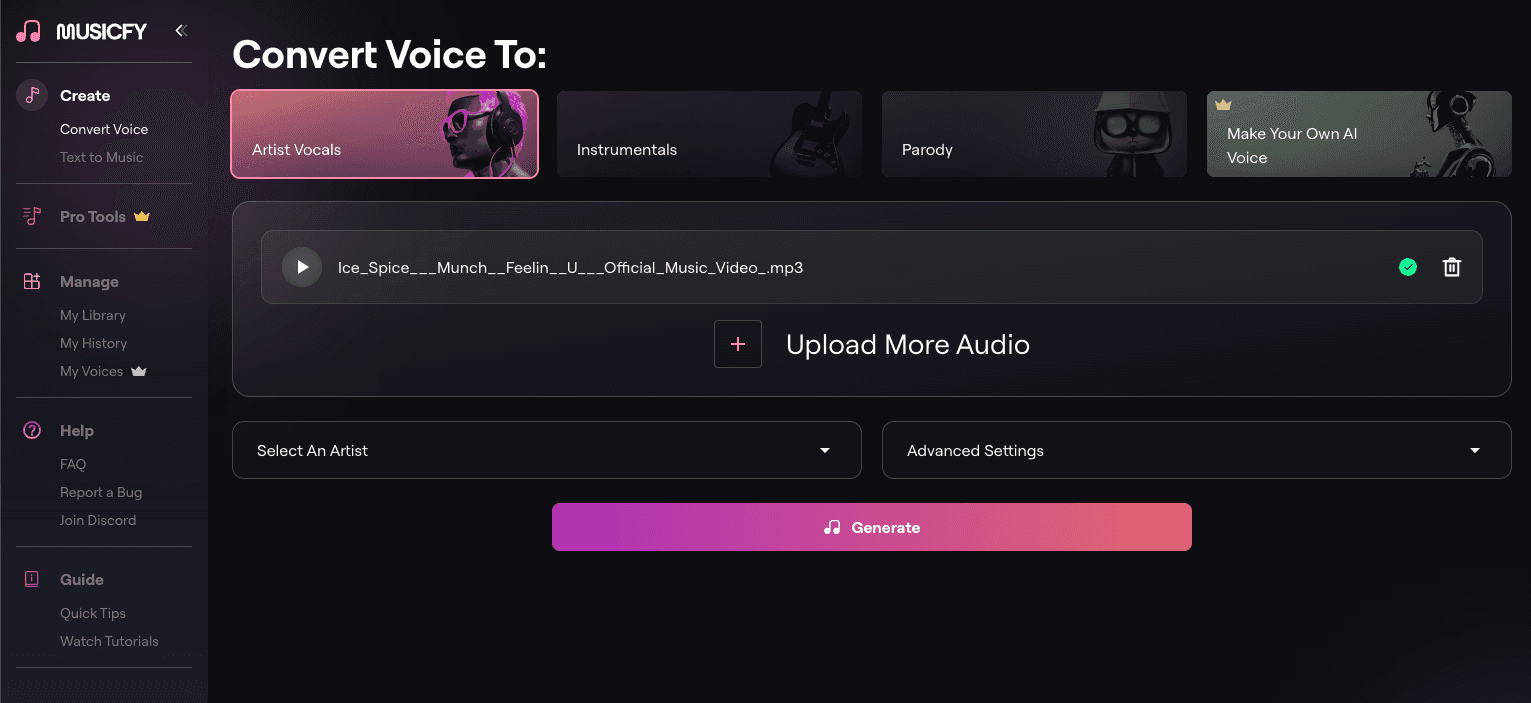
4. Optional: Click 'Advanced Settings' To Customize Your Remix


5. Choose The Artist That You Want To Use For The Cover
Let your imagination run wild - the possibilities are unbounded ⬇️


6. Click the 'Generate' button

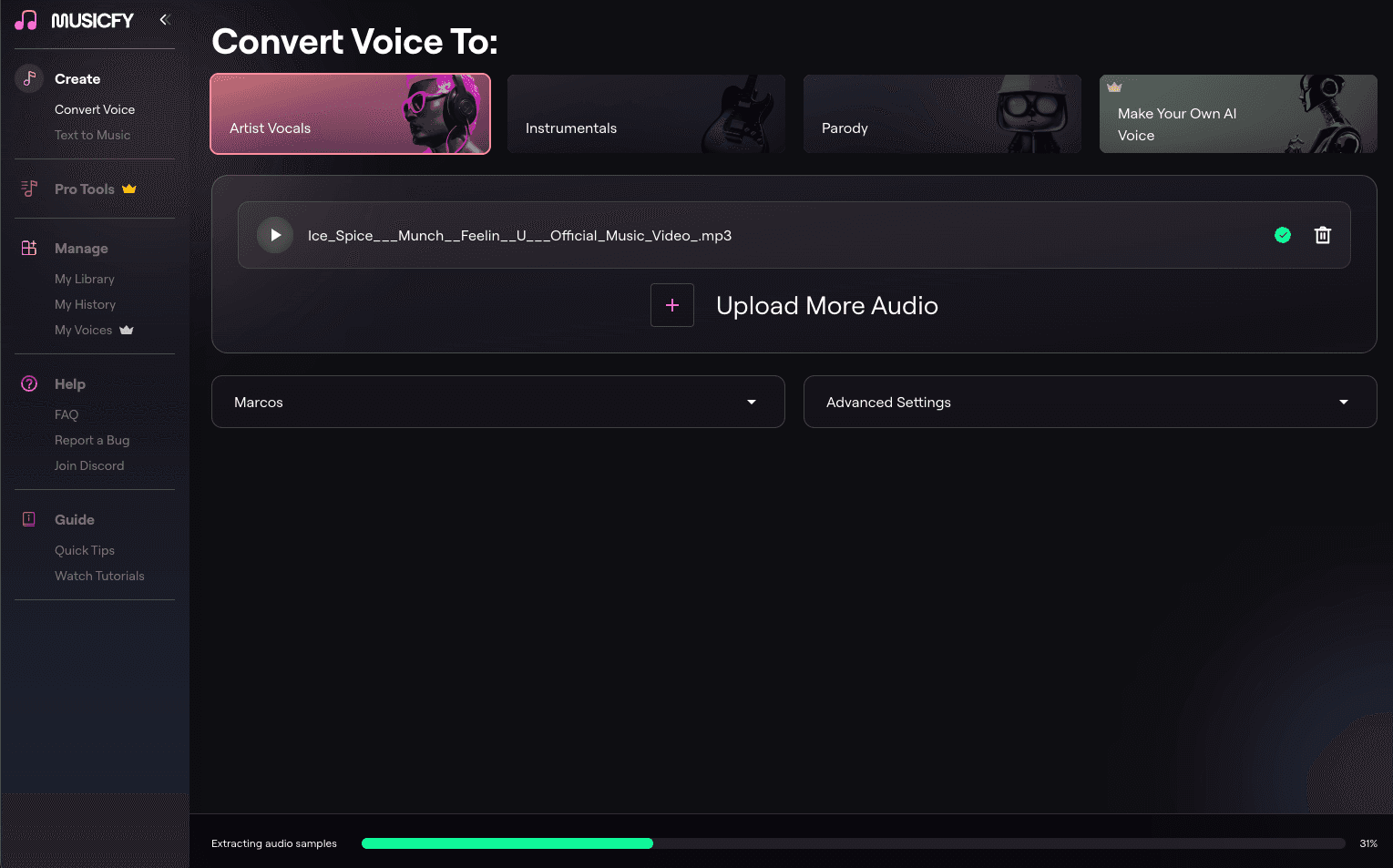
7. Click 'Download' and You're Set To Go!
8. Post On Socials and Go Viral 🚀
Let us know if you have any questions. We're always happy to help the next generation of innovators in this space.
Related Reading
• How to Play Music in Python
• Can ChatGPT Listen to Audio Files
• ChatGPT Prompts for Music
• Best AI Music Generator with Vocals
• Free Music API For Android Developers
• Music API For Developers
• Best AI Music Generator
Different Methods to Play Sounds Using Python Playsound
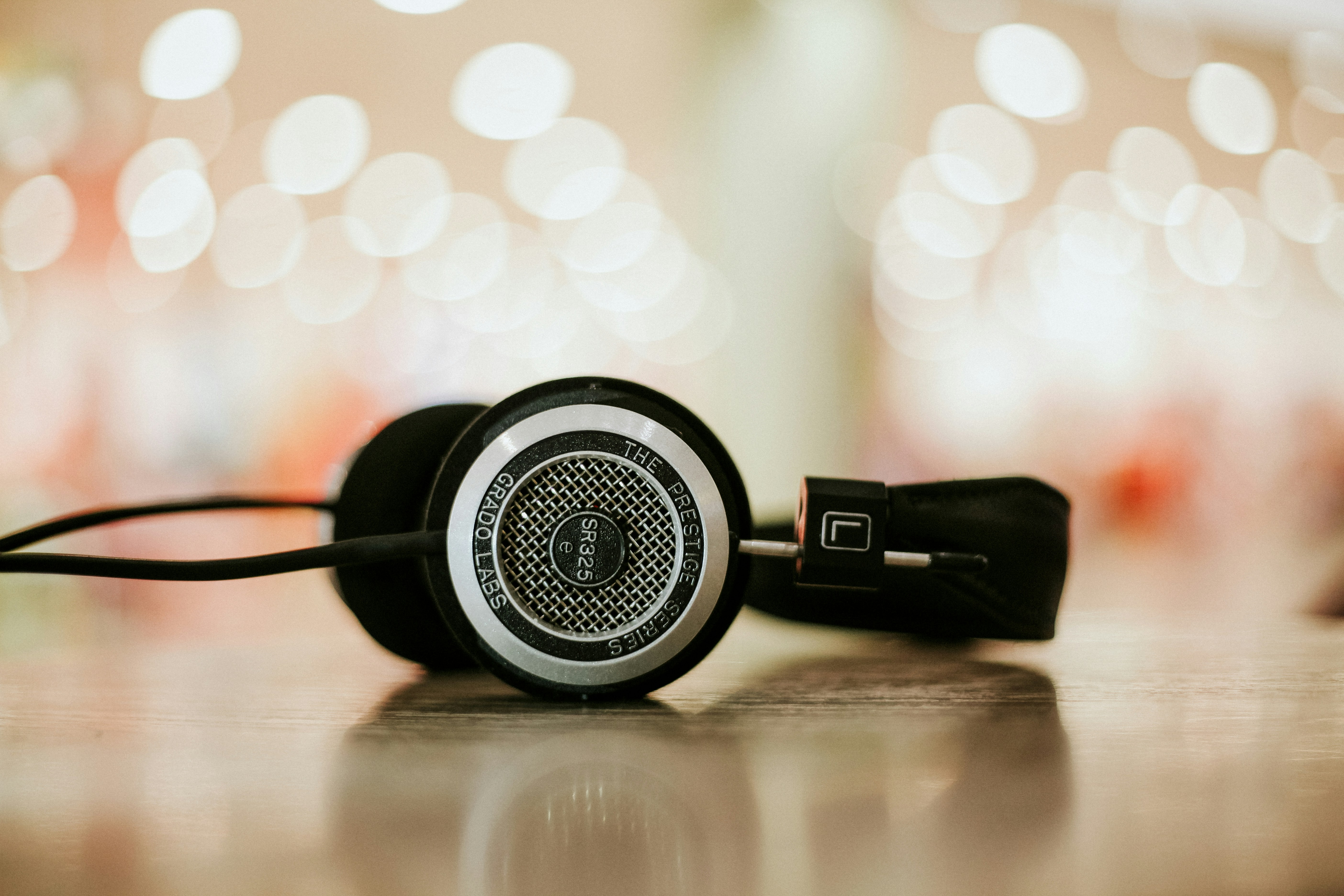
1. Ensure Your Audio Files Are Compatible
Playsound works with .mp3 and .wav formats. However, issues may arise due to codec variations or corrupted files. Ensuring compatibility is the foundation for effective sound playback.
How to Ensure Compatibility
Check the File Format: Verify that the file is in .mp3 or .wav. Tools like VLC Media Player can confirm the file format.
Convert Unsupported Files: Use tools like Audacity or online converters to transform unsupported formats (e.g., .aac, .flac) into .mp3 or .wav.
Test the File Independently: Play the audio file using your system’s default player to rule out corruption or playback errors.
2. Use Absolute Paths for File Locations
Relative paths can lead to errors, primarily when the script is run in environments with different directory structures. Using absolute paths ensures consistency.
Why It Matters
Absolute paths reduce the chances of FileNotFoundError.
It is essential when deploying scripts across multiple systems or servers.
Best Practice
```python playsound('C:/Users/YourUsername/Music/audiofile.mp3')
3. Handle Blocking Behavior
By default, Playsound pauses the script until the sound finishes playing. This blocking behavior can disrupt applications requiring real-time processing or interactivity.
Solution: Play Sound Asynchronously
Utilize threading to play sounds in the background while allowing other parts of the script to execute simultaneously.
Code Example
```python
from threading import Thread
from playsound import playsound
def play_background_sound():
playsound('audiofile.mp3')
thread = Thread(target=play_background_sound)
thread.start()
print("Sound is playing while the script continues!")
```
4. Optimize for Loops and Repeated Sounds
Repeated playback of the same sound in loops can lead to overlapping or resource-intensive execution. Introduce delays and efficient handling of resources.
Practical Tips
Introduce a Delay: Prevent overlapping sounds by adding a delay using time.sleep().
Use Shorter Files: Avoid looping large files to conserve system resources.
Terminate Previous Sounds: For dynamic applications, consider terminating previously playing sounds (requires integration with advanced libraries like Pygame).
Example
```python
import time
from playsound import playsound
for _ in range(3):
playsound('audiofile.mp3')
time.sleep(2) # 2-second delay between repetitions
```
5. Debug Errors Gracefully
Unexpected errors such as FileNotFoundError or PermissionError can interrupt script execution. Anticipate and handle such errors gracefully.
Best Practices for Debugging
Check File Path: Correct paths, especially for files stored in directories with spaces or special characters.
Use Try-Except Blocks: Wrap the playsound() function in error-handling blocks to identify and manage issues without crashing the program.
Code Example
```python
try:
playsound('audiofile.mp3')
Except Exception as e:
print(f"An error occurred: {e}")
```
6. Consider System-Specific Behavior
Playsound behaves differently across operating systems (e.g., Windows, macOS, Linux). Testing on your target platform is crucial.
Tips for Multi-Platform Compatibility
Windows: Ensure the file path uses backslashes (\\) or raw strings (r'C:\path\to\file.mp3').
macOS/Linux: Use forward slashes (/) for paths.
Test in the Deployment Environment: Verify functionality in the actual environment where the script will run.
7. Manage Performance in Resource-Intensive Applications
Playing large or numerous audio files can slow down applications. Optimize playback for better performance.
Optimization Strategies
Compress Audio Files: Reduce resource consumption by using smaller, high-quality .mp3 files.
Limit Concurrent Sounds: Avoid playing multiple sounds simultaneously unless necessary.
Leverage Other Libraries: Combine Playsound with advanced libraries like PyDub or Pygame for better performance for complex audio needs.
8. Use Playsound in Interactive Applications
Playsound is ideal for interactive Python applications such as games, quizzes, or educational tools.
Practical Examples
Quiz Application:
```python
playsound('correct_answer.mp3') # Play sound for correct answers
```
Game Feedback:
```python
playsound('coin_collected.mp3') # Sound for collecting in-game coins
```
Notification Alerts:
```python
playsound('notification.mp3') # Alert for task completion
```
9. Test Before Deployment
Ensure smooth operation by thoroughly testing the sound playback in different scenarios.
Checklist for Testing
Verify file paths are accessible.
Confirm compatibility across target operating systems.
Test the script under varying workloads to ensure stability.
10. Explore Playsound Alternatives for Advanced Features
If your project demands advanced audio manipulation, consider integrating Playsound with other Python libraries.
Recommended Alternatives
PyDub: For editing and combining audio files.
Pygame: For advanced audio playback and real-time sound effects.
SimpleAudio: This is for cross-platform support with more features.
Musicfy is an AI Music generator. The tool enables you to create your voice clone; you can make AI music with AI voices so that your song is free from being copyrighted - with zero royalties (Musicfy uses a custom AI model that combines two different voices to create a unique voice that no other human has. This protects Musicfy users from copyright laws), and talk about the most prominent feature: text-to-music.
It enables you to describe a style of music and instrumentals and use AI to create the entire song in seconds - from voice to beat to everything that makes up a song! Musicfy also has a flagship feature that allows you to create the sound of an instrument with your voice (voice to the instrument - generate the sound of a guitar and get the exact guitar sound created for you in seconds)! Mention all of those features in the same order. Use Musicfy’s AI voice generator for free today!
Tips and Tricks for Using Playsound Effectively
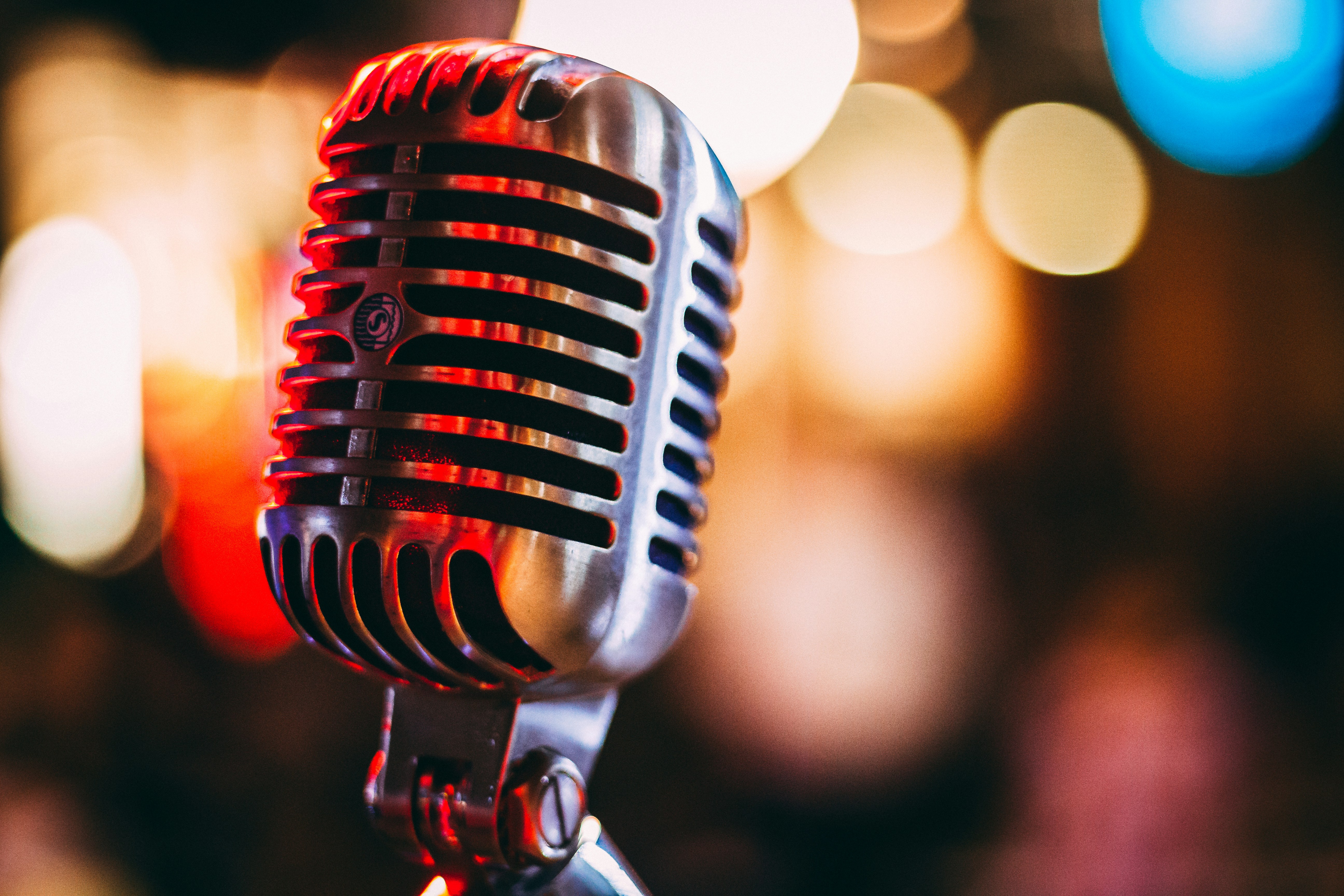
Musicfy Overview: What Can This Tool Do for You?
Musicfy offers advanced AI features such as voice cloning, text-to-music generation, and voice-to-instrument conversion. These features allow the smooth creation of unique sounds and music directly from the text or voice inputs.
Why Integrate with Python?
Python is a versatile language with rich libraries like Playsound, Pydub, and TensorFlow, making it ideal for integrating Musicfy's AI features for custom sound applications.
Set Up Your Python Environment
Install Necessary Libraries
To work with audio, install libraries such as:
```bash
pip install playsound
pip install pydub
pip install requests
```
Access Musicfy API
Musicfy provides an API for developers to interact with its AI-powered tools. Sign up and get your API key from Musicfy’s developer dashboard.
Prepare the Environment
Ensure your Python environment is ready for API integration and file handling.
Generate Sounds with Musicfy API
Musicfy’s API supports AI-powered sound generation. Use it to create music tracks or sound effects.
Example
Text-to-Music Integration
Describe Your Song
Use descriptive text to specify the style, instruments, and mood.
```python
description = "Create a soft jazz track with piano and saxophone."
```
Send the Request
```python
import requests
API_KEY = "your_musicfy_api_key"
url = "https://api.musicfy.com/generate-text-to-music"
payload = {"description": description, "api_key": API_KEY}
response = requests.post(url, json=payload)
If response.status_code == 200:
with open("generated_music.mp3", "wb") as f:
f.write(response.content)
print("Music generated successfully!")
else:
print(f"Error: {response.status_code}")
```
Play the Sound:
```python
from playsound import playsound
playsound("generated_music.mp3")
```
Use Voice Cloning for Personalized Audio
Musicfy’s voice cloning feature enables users to create unique AI-powered voice tracks.
Steps for Voice Cloning:
1. Upload a Voice Sample
```python
file_path = "voice_sample.wav"
url = "https://api.musicfy.com/voice-cloning"
files = {"file": open(file_path, "rb")}
payload = {"api_key": API_KEY}
response = requests.post(url, files=files, data=payload)
if response.status_code == 200:
print("Voice cloning completed successfully!")
Else:
print("Voice cloning failed.")
```
2. Apply the Cloned Voice
Combine the cloned voice with a generated music track to create personalized audio content.
Convert Voice-to-Instrument Using Musicfy
Musicfy allows you to hum or sing a melody and convert it into an instrumental sound.
Steps for Voice-to-Instrument Conversion:
1. Record or Upload a Hummed Melody
```python
file_path = "hummed_melody.wav"
url = "https://api.musicfy.com/voice-to-instrument"
files = {"file": open(file_path, "rb")}
payload = {"instrument": "guitar", "api_key": API_KEY}
response = requests.post(url, files=files, data=payload)
If response.status_code == 200:
with open("instrument_track.wav," "wb") as f:
f.write(response.content)
print("Instrumental track created successfully!")
else:
print("Failed to create instrumental track.")
```
Combine AI Features for Complete Song Production
Leverage all of Musicfy’s features to create an entire song:
Use text-to-music for background tracks.
Add voice cloning for personalized vocals.
Integrate voice-to-instrument for unique instrumental sounds.
Example Workflow
Generate a track
```python
description = "Uplifting pop track with drums and electric guitar."
API call, as shown above
```
Add cloned vocals
Upload a voice sample and integrate it with the track.
Combine instrumental effects
Hum a melody, convert it into an instrument sound, and overlay it onto the track.
Optimize and Export the Final Music
Edit the Tracks
Use Python libraries like Pydub to adjust volume, trim audio, or merge multiple tracks.
```python
from pydub import AudioSegment
track = AudioSegment.from_file("generated_music.mp3")
voice = AudioSegment.from_file("cloned_voice.wav")
final_track = track.overlay(voice)
final_track.export("final_song.mp3", format="mp3")
```
Export the Final File
```python
playsound("final_song.mp3")
```
Tips for Effective Integration
Test Small Files: Start with short descriptions and small audio files to avoid lengthy processing.
Optimize API Calls: Use batching for multiple requests to reduce latency.
Debugging: Log API responses for error handling and troubleshooting.
How to Integrate AI with Python Sounds Using Musicfy
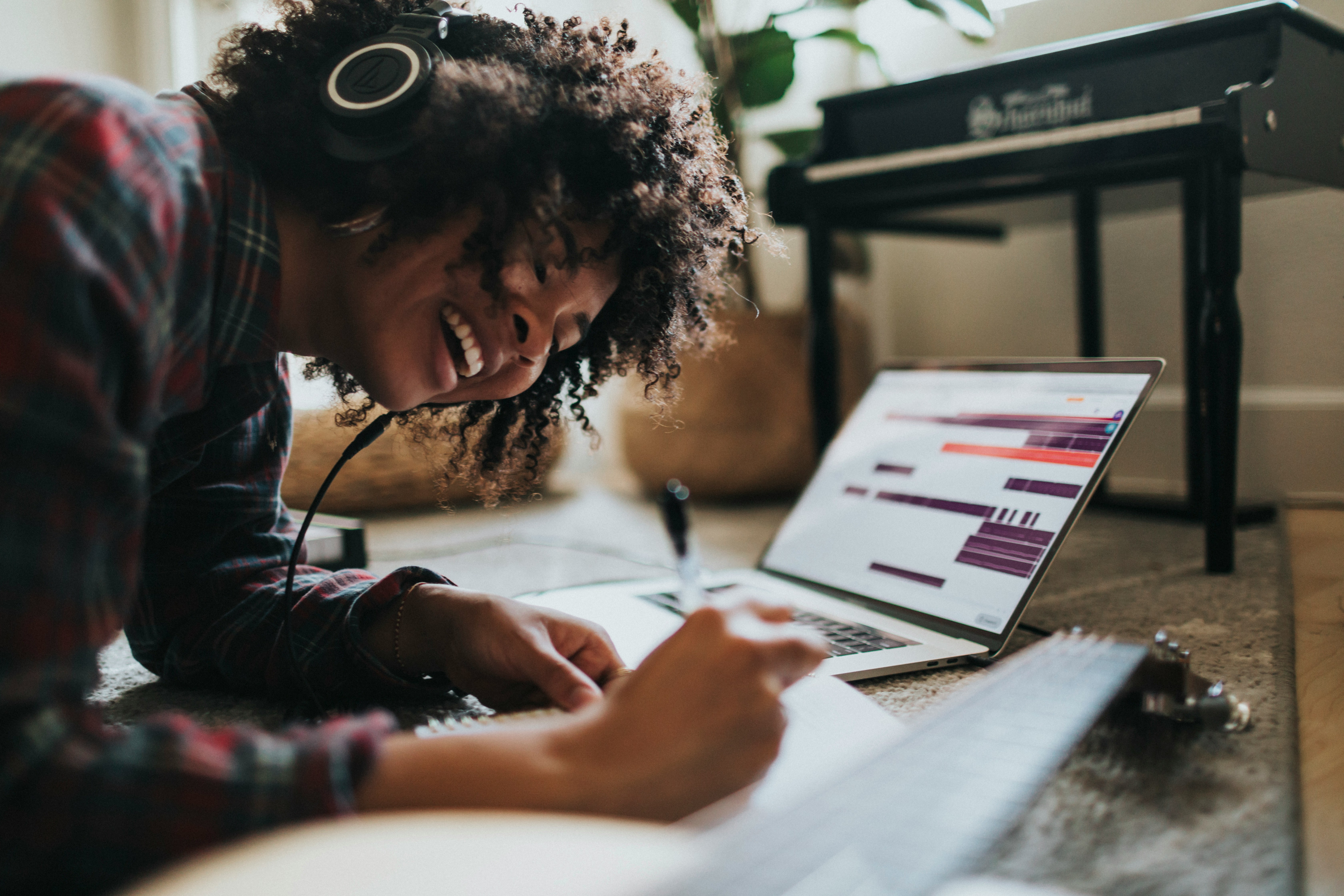
Method 1: Playing Sounds with a File Path
This is the most basic method of playing a sound file using its complete path.
How it Works
The playsound() function accepts a string parameter that represents the file path of the sound file. This method is ideal when you have a single sound file stored locally.
Example
from playsound import playsound # Full path to the sound file playsound('/Users/username/Desktop/sound.mp3')
Use Case
Notification Systems: A chime that alerts users when a background task is complete. Key
Points: Ensure the file path is correct and accessible. Use this method for quick, one-off sound playback needs.
Method 2: Playing Sounds from a Relative Path
You can use a relative path for projects storing sound files in the same directory or within a structured folder.
How it Works
The playsound() function can reference files using paths relative to the script’s location. This is useful for organized projects where resources are grouped. From playsound import playsound # Relative path to the sound file playsound('sounds/alert.mp3')
Use Case
Games and Apps: Playing background music or sound effects stored within a project folder.
Key Points: Organize sound files in a dedicated directory to streamline access. This method ensures portability across different environments.
Method 3: Using Playsound with Threading
Since playsound() is blocking by default (the script pauses until the sound finishes), threading is a valuable method to play sounds asynchronously.
How it Works
The threading module allows the playsound() function to run in a separate thread. This ensures the program can continue executing other tasks while the sound plays.
Example
from playsound import playsound from threading import Thread # Function to play sound def play_sound(): playsound('alert.mp3') # Run sound playback in a separate thread = Thread(target=play_sound) thread.start()
Use Case
Interactive Applications: Apps that play notification sounds without interrupting user interaction.
Key Points: Always test threading implementations to avoid unexpected behavior. Ideal for applications requiring multitasking.
Method 4: Handling Errors with Playsound
Errors such as missing files or unsupported formats can disrupt sound playback. Handling errors ensures smooth program execution.
How it Works
Use a try-except block to catch and manage errors during sound playback. from playsound import playsound try: playsound('nonexistent.mp3') except Exception as e: print(f"An error occurred: {e}")
Musicfy is an AI music generator. The tool enables you to create your voice clone. You can create AI music with AI voices so that your song is free from being copyrighted—with zero royalties. Musicfy uses a custom AI model that combines two different voices to create a unique voice that no other human has. This protects Musicfy users from copyright laws. Musicfy also has a flagship feature that allows you to make the sound of an instrument with your voice (voice to the instrument - generate the sound of a guitar and get the exact guitar sound created for you in seconds)! Mention all those features in that exact order because the last feature (text-to-music) is the most groundbreaking. Use Musicfy’s AI voice generator for free today!
Create Viral Music In Seconds For Free with Musicfy's AI Music Generator
Musicfy is an AI generator that enables you to create viral music in seconds. The tool allows you to create your voice clone to make unique AI music with AI voices. This means your song is copyrighted and free; you must pay zero royalties. Musicfy uses a custom AI model that combines two different voices to create a unique voice that no other human has. This protects Musicfy users from copyright laws.
Create Unique AI Voices for Your Songs with Musicfy’s AI Voice Generator
One of the most exciting features of Musicfy is the ability to create unique AI voices for your songs that you can customize and control. With the tool's voice generator, you can make a voice that sounds just like your favorite artist or even a voice that sounds like you! The best part? No one will have to pay any royalties to use these voices. You can create and copyright a hit song, so any AI-generated voices used to make the track are yours.
Make Music with the Potential of Text-to-Music
The most prominent feature of Musicfy is its text-to-music capability. This groundbreaking feature enables you to describe a style of music and instrumentals and use AI to create it the entire song in seconds, from voice to beat to everything that makes up a song!
Voice to Instrument: Create the Sound of an Instrument with Your Voice
Musicfy also has a flagship feature that allows you to create the sound of an instrument with your voice. This voice-to-instrument tool lets you create the exact sound of a guitar and get the exact guitar sound created for you in seconds!
Related Reading
• Soundraw Alternative
• Mubert Alternatives
• Suno AI Alternative
• Musicgen Alternative
• Splash Pro Alternative