You might be surprised to learn about music's role in artificial intelligence. For example, various AI programs can generate music from scratch, create musical scores from written prompts, and even imitate the styles of specific artists.
Considering this, it’s natural to wonder: Can ChatGPT make music? The answer is yes! In fact, with the proper coding knowledge, you can use ChatGPT to help you create music in Python. This guide will teach you how to play music in Python so you can start your musical journey with AI today.
One way to simplify learning how to play music in Python is to use an AI tool like Musicfy’s AI voice generator. This program allows you to create a song with vocals in minutes. You only need to enter a few prompts; the tool will generate a song for you.
If you can't wait to use Musicfy's Free AI Voice Generator, you can try out 1000+ celebrity voices, like:
You can use all of these voices and 1000+ more for free today on create.musicfy.lol!
Table Of Contents
Complete Step-by-Step Guide On How To Use Musicfy's AI Voice Generator
How to Integrate AI Music Tools with Python to Play and Manipulate Sounds
Create Viral Music In Seconds For Free with Musicfy's AI Music Generator
Setting Up Your Python Environment
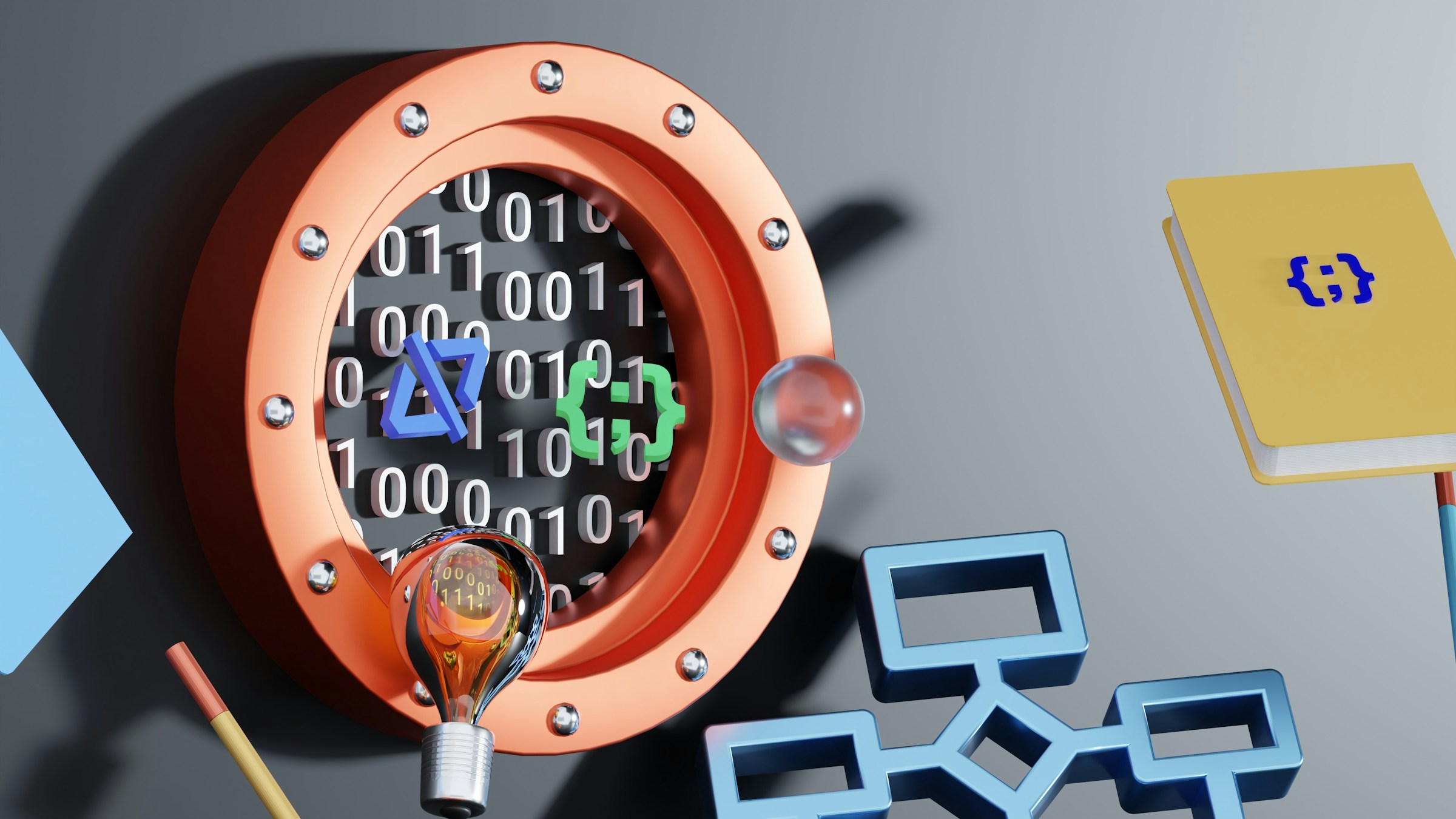
Install Python for Music Playback in Python
Python is the foundation of this guide, and ensuring you have the correct version installed is the first step.
Download Python
Visit python.org and download the latest version of Python (Python 3.6 or later is recommended for compatibility with most audio libraries).
Install Python
Follow the installation wizard for your operating system. Ensure you check the box "Add Python to PATH" during installation, as this makes Python accessible from the command line.
Verify Installation
Open a terminal or command prompt and type:
bash
python --version
You should see the installed Python version. If not, revisit the installation steps.
Install Required Libraries for Playing Music in Python
Python has a rich ecosystem of libraries that simplify audio playback and manipulation. This guide will use three popular libraries: pygame, playsound, and pydub.
Install Libraries Using Pip
Open your terminal or command prompt and run:
bash
pip install pygame playsound pydub
What Each Library Does
pygame: A versatile game development library with robust audio playback capabilities.
playsound: A lightweight library for playing audio files with minimal setup.
pydub: A library for manipulating audio (e.g., trimming, changing volume) and playing it.
Verify Installation
Confirm the libraries are installed by importing them in Python:
python
import pygame
import playsound
import pydub
print("Libraries installed successfully!")
Prepare Your Music Files Before Playing Music in Python
Before running your Python script, ensure your audio files are properly formatted and accessible.
File Format Compatibility
playsound: Best for .mp3 files.
pygame: Works well with .wav and .ogg formats.
pydub: Supports multiple formats (.mp3, .wav, .ogg), but may require additional software for some formats.
Convert Audio Files If Necessary:
Use a free tool like Audacity or an online converter to change file formats if needed.
File Placement
Place your audio file in the same directory as your Python script to avoid path-related errors. Alternatively, use absolute paths to specify file locations.
Naming Convention
Use simple, descriptive names for your audio files (e.g., song1.mp3) to prevent typos and confusion.
Install Additional Dependencies for Playing Music in Python (Optional)
Some libraries require additional system-level dependencies for advanced functionality.
For pydub
Install FFmpeg, a robust multimedia framework used for audio processing.
Follow these steps:
Download FFmpeg from ffmpeg.org.
Add the FFmpeg executable to your system PATH.
Verify FFmpeg Installation
Open a terminal or command prompt and type:
bash
ffmpeg -version
You should see the FFmpeg version information.
Test Your Environment for Playing Music in Python
Before diving into programming, ensure everything is set up correctly by running a simple script.
Create a Test Script
Open a text editor or IDE (e.g., VS Code, PyCharm) and create a new file (test_audio.py).
Paste the following code:
python
from playsound import playsound
Test playsound
playsound('test_audio.mp3')
print("Audio played successfully!")
Replace test_audio.mp3 with the name of your audio file.
Run the Script
Navigate to your script directory in the terminal and run:
bash
python test_audio.py
If you hear the audio playback, your environment is ready.
Related Reading
• AI Music Prompt
• How Does AI Music Work
• ChatGPT Music Composition
• ChatGPT Music Generation
• Python Playsound
• How Is AI Music Made
Complete Step-by-Step Guide On How To Use Musicfy's AI Voice Generator
Just a reminder, if you can't wait and are eager to use Musicfy's Free Spongebob AI Voice Generator, you can try out our free Spongebob Squarepants AI voice generator, and 100+ more celebrity voices and popular voices on create.musicfy.lol for free right now!
Video Guide
Written Guide
1. Download An Audio File or Find A Youtube Link For A Song That You Want To Use for A Voice Over or An AI Song Cover
2. Go To Create.musicfy.lol
You will land on this page

3. Upload Your Audio File
You can upload the audio file, or you can upload a Youtube link
Upload Audio File:

Upload Youtube Link:

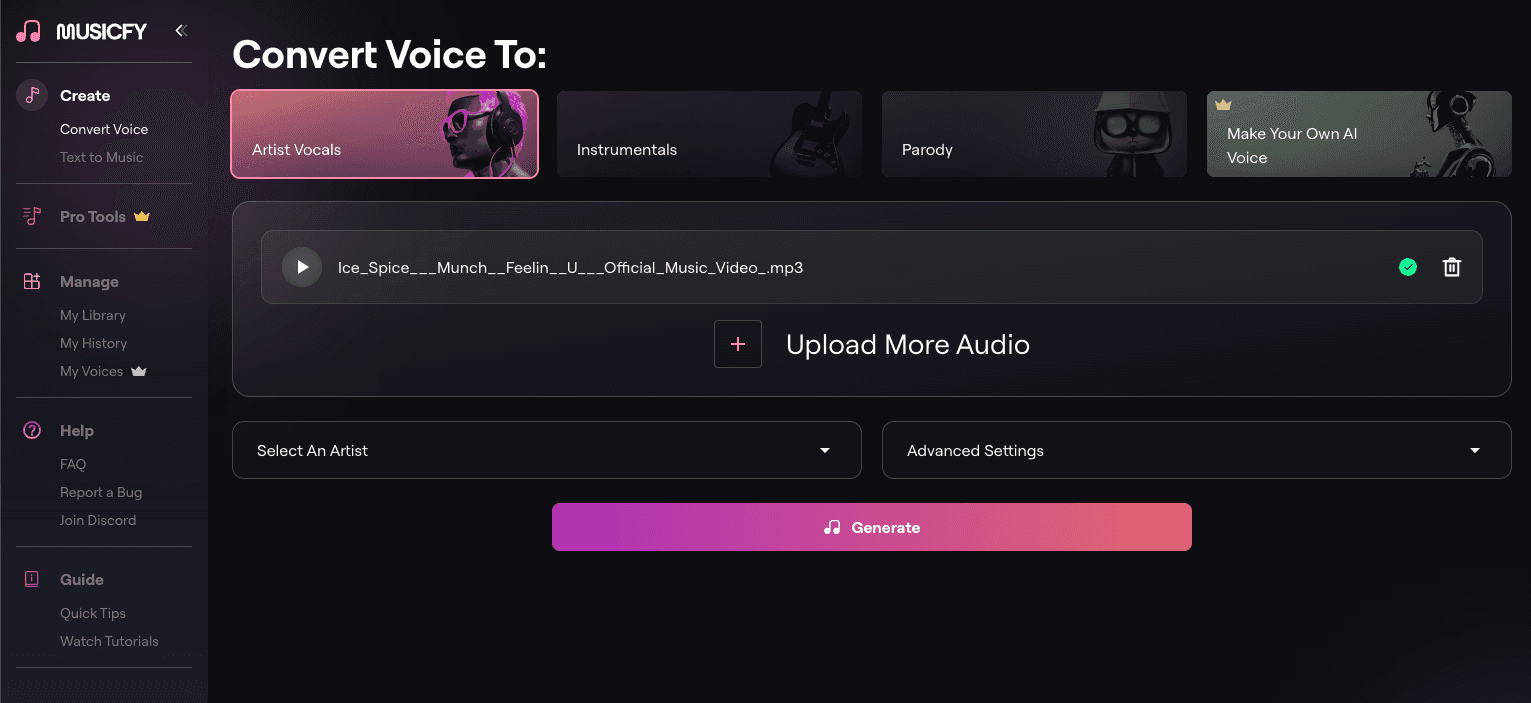
4. Optional: Click 'Advanced Settings' To Customize Your Remix


5. Choose The Artist That You Want To Use For The Cover
Let your imagination run wild - the possibilities are unbounded ⬇️


6. Click the 'Generate' button

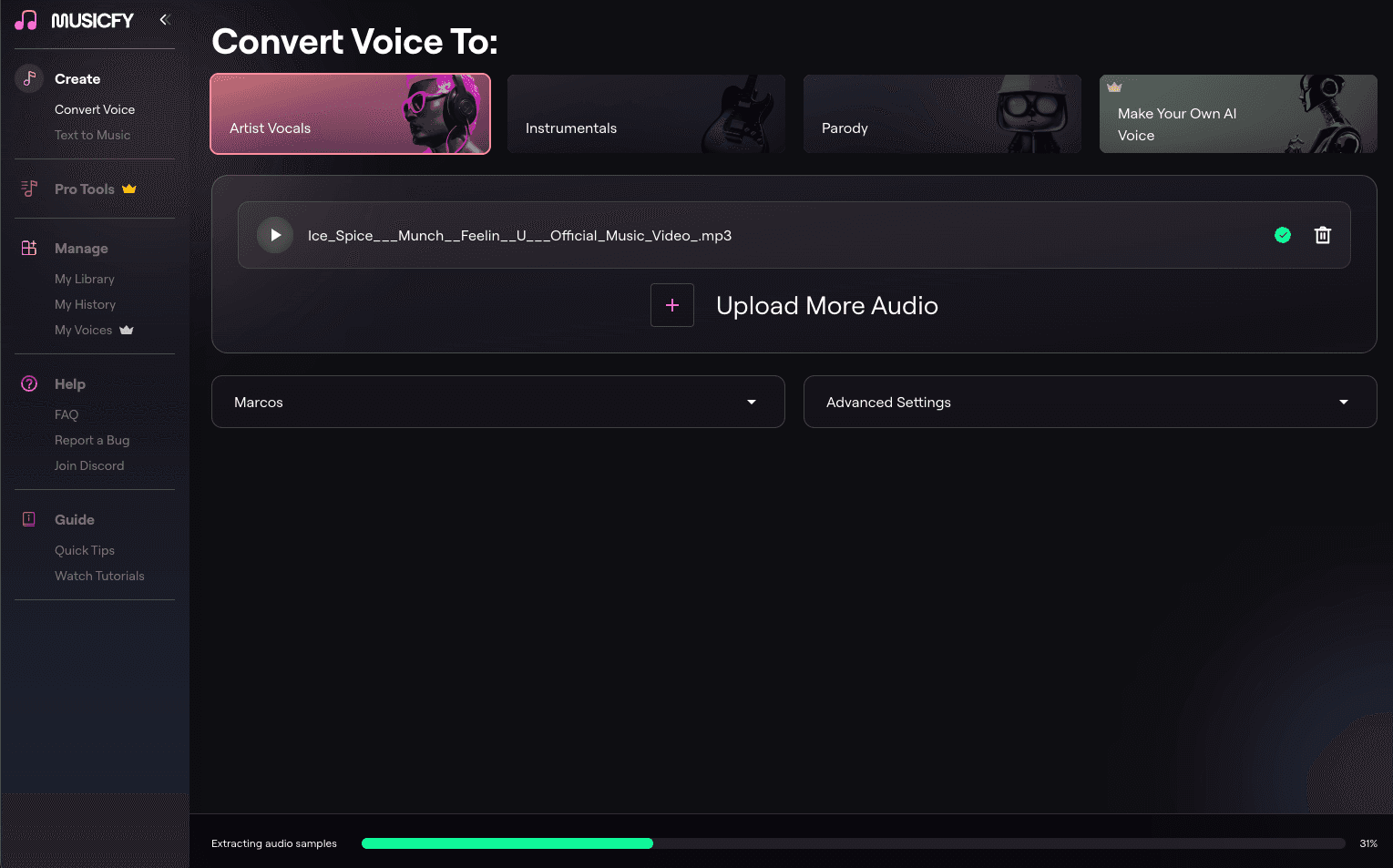
7. Click 'Download' and You're Set To Go!
8. Post On Socials and Go Viral 🚀
Let us know if you have any questions. We're always happy to help the next generation of innovators in this space.
Related Reading
• Can ChatGPT Listen to Audio Files
• ChatGPT Prompts for Music
• Best AI Music Generator with Vocals
• Free Music API For Android Developers
• Music API For Developers
• Best AI Music Generator
Playing Music with Python
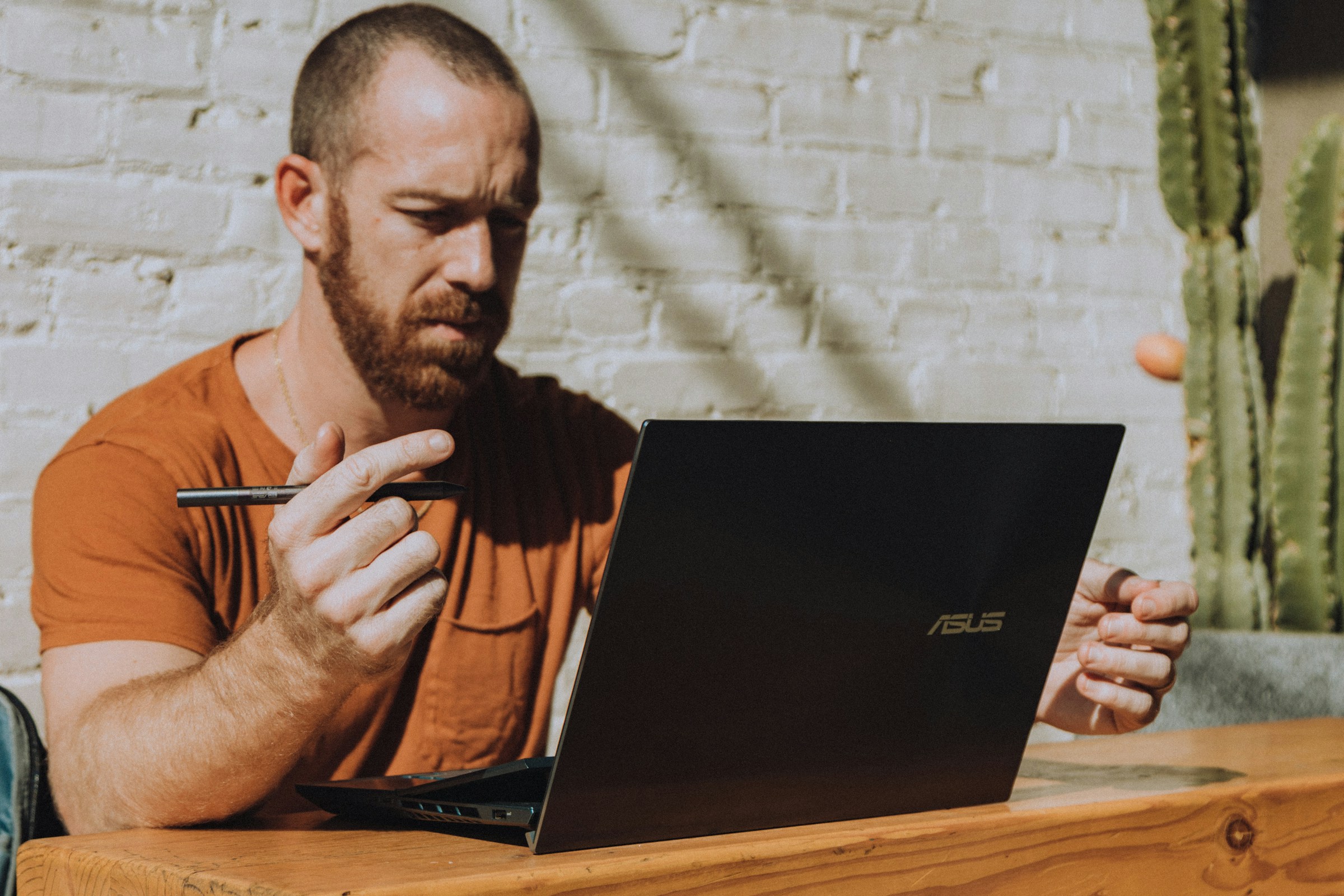
Play Your First Tune with Playsound
The playsound library is one of the simplest ways to play audio files in Python. It’s ideal for quick playback with minimal configuration.
Key Features
Supports .mp3 and .wav formats.
Requires no additional setup beyond installation.
Example Code
python
from playsound import playsound
Play an audio file
playsound('your_audio_file.mp3')
How It Works
Replace 'your_audio_file.mp3' with the name of your audio file. The playsound function blocks the execution of the script until the audio finishes playing.
When to Use It
This is for straightforward audio playback without needing advanced controls like pausing or looping.
Kick it Up a Notch with Pygame.
The pygame library is more versatile and offers greater control over audio playback. It supports formats like .wav and .ogg.
Key Features
Play, pause, stop, and loop audio files.
Ideal for applications requiring more interaction, such as games.
Example Code
Python
import pygame
Initialize the mixer module
pygame. mixer.init()
Load and play the audio file
pygame.mixer.music.load('your_audio_file.wav')
pygame.mixer.music.play()
Keep the script running until the music finishes
while pygame.mixer.music.get_busy():
pass
How It Works
`pygame.mixer.init()` initializes the audio mixer.
`pygame.mixer.music.load()` loads the audio file.
`pygame.mixer.music.play()` starts playback. The while loop ensures the script doesn’t terminate until the audio stops.
Additional Controls
Pause Playback:
python
pygame.mixer.music.pause()
Resume Playback:
python
pygame.mixer.music.unpause()
Stop Playback:
python
pygame.mixer.music.stop()
When to Use It
This is for audio control projects, such as games or interactive applications.
Get Creative with Pydub
The pydub library is excellent for manipulating audio files and is compatible with various formats. It becomes a powerful tool paired with simple audio (a playback library).
Key Features
Adjust volume, trim audio, or merge files.
Supports .mp3, .wav, and more.
Example Code
python
from pydub import AudioSegment
from pydub.playback import play
Load the audio file
audio = AudioSegment.from_file('your_audio_file.mp3')
Play the audio
play(audio)
How It Works
`AudioSegment.from_file()` loads the audio file.
`play()` starts playback.
Advanced Manipulations
Trim Audio:
python
trimmed_audio = audio[:10000] # Keep only the first 10 seconds
play(trimmed_audio)
Change Volume
python
louder_audio = audio + 10 # Increase volume by 10 dB
play(louder_audio)
Merge Audio Files
python
audio2 = AudioSegment.from_file('another_audio_file.mp3')
merged_audio = audio + audio2 # Combine two audio files
play(merged_audio)
When to Use It
This is for advanced projects requiring audio manipulation before playback.
Tips for Choosing the Right Library
Use playsound: If you need a quick, simple solution for playing .mp3 files.
Use pygame: For projects requiring playback controls like pause, resume, or loop.
Use pydub: If you want to manipulate audio files (e.g., trimming, merging, or changing volume) before playback.
Error Handling
Audio playback might encounter errors due to missing files or unsupported formats. Use try-except blocks to handle these issues gracefully:python
try:
playsound('your_audio_file.mp3')
except Exception as e:
print(f"An error occurred: {e}")
Why Use Musicfy?
Musicfy is an AI music generator that enables you to create your voice clone. You can create AI music with AI voices so that your song is free from being copyrighted with zero royalties. Musicfy uses a custom AI model that combines two different voices to create a unique voice that no other human has. This protects Musicfy users from copyright laws. The tool’s most prominent feature is text-to-music.
It enables you to describe a style of music and instrumentals and use AI to create the entire song in seconds—from voice to beat to everything that makes up a song! Musicfy also has a flagship feature that allows you to create the sound of an instrument with your voice. With voice-to-instrument, you can create the sound of a guitar and get the exact guitar sound created for you in seconds! Start using Musicfy’s AI voice generator for free today!
How to Integrate AI Music Tools with Python to Play and Manipulate Sounds

Integrating AI music tools with Python unlocks countless opportunities for automated music creation, playback, analysis, and experimentation. For example, you can write Python scripts to automatically play tracks created by AI music generators, modify their audio properties (like tempo and pitch), or even automate downloading and organizing files. With the proper programming knowledge, you can build custom applications that combine AI music tools with your unique functionalities.
Examples of AI Music Tools You Can Integrate
You can combine a wide variety of AI music generators with Python applications. For example, Musicfy is a groundbreaking AI music generator that creates unique compositions, voice clones, and instrumentals. Features like text-to-music allow you to describe a music style and generate a whole track in seconds. OpenAI Jukebox is another AI that produces music in various genres and styles, including lyrics.
It outputs raw audio that can be played or further processed in Python. Magenta (by Google) focuses on music generation and manipulation using machine learning. It generates MIDI files and audio compositions. Amper Music and AIVA are cloud-based platforms that create AI-driven compositions, which can be integrated into Python projects via APIs.
Steps to Integrate AI Music Tools with Python
Step 1: Access the AI Music Tool
Using Musicfy: Sign up for Musicfy and use its features to generate music. For automation, use Musicfy's API (if available) to connect directly to Python. Download generated tracks for playback or manipulation.
Using APIs: Many AI tools like Magenta or Amper provide APIs to interact programmatically. Install the API's SDK or use Python's requests library to send and receive data.
Example (using a fictional Musicfy API)
python
import requests
API endpoint and parameters
url = "https://api.musicfy.com/generate"
params = {
"style": "jazz",
"instrument": "piano",
"duration": 60 # in seconds
}
Make API request
response = requests.post(url, json=params)
Save the generated music file
with open("generated_music.mp3", "wb") as file:
file.write(response.content)
print("Music generated and saved as 'generated_music.mp3'")
Step 2: Play the Generated Music in Python
Once you've generated or downloaded music using the AI tool, use Python libraries to play the file.
Using pygame:
python
import pygame
Initialize pygame mixer
pygame.mixer.init()
Load and play the music file
pygame.mixer.music.load("generated_music.mp3")
pygame.mixer.music.play()
Keep the program running while the music plays
while pygame.mixer.music.get_busy():
pass
Using playsound
python
from playsound import playsound
Play the music file
playsound("generated_music.mp3")
Step 3: Manipulate the Music
Python libraries like pydub or librosa can be used to modify AI-generated audio.
Changing the Speed
python
from pydub import AudioSegment
Load the music file
audio = AudioSegment.from_file("generated_music.mp3")
Speed up the audio
faster_audio = audio.speedup(playback_speed=1.5)
Save the modified audio
faster_audio.export("faster_music.mp3", format="mp3")
print("Modified audio saved as 'faster_music.mp3'")
Adding Effects (Echo)
python
from pydub import AudioSegment
from pydub.effects import echo
Load the audio file
audio = AudioSegment.from_file("generated_music.mp3")
Apply echo effect
echoed_audio = echo(audio)
Save the modified file
echoed_audio.export("echoed_music.mp3", format="mp3")
Step 4: Build a Custom Application
Use Python frameworks to create applications that integrate AI music tools:
Interactive Music Player with tkinter: Build a GUI for playing and manipulating AI-generated music.
python
import tkinter as tk
from playsound import playsound
def play_music():
playsound("generated_music.mp3")
root = tk.Tk()
root.title("Musicfy Player")
play_button = tk.Button(root, text="Play Music", command=play_music)
play_button.pack()
root.mainloop()
Music Generator with Input Prompts: Combine AI tools and Python to create a prompt-based music generator.
python
import requests
prompt = input("Describe the music style (e.g., upbeat jazz with piano): ")
response = requests.post("https://api.musicfy.com/generate", json={"style": prompt})
With open("custom_music.mp3", "wb") as file:
file.write(response.content)
print("Your custom music has been generated!")
Benefits of Integrating AI Music Tools with Python
Integrating AI music generators with Python opens a world of music composition and sound design possibilities. For instance, you can automate repetitive tasks like downloading and processing music files to save time. You can also build applications tailored to your needs, such as music players or real-time soundboards. You can generate large batches of music or sounds using APIs for more significant projects. Finally, combining Python's flexibility with AI's creativity can help you explore new possibilities in music production.
Expanding Integration Possibilities
You can expand the possibilities of integrating AI music tools and Python in various ways. For example, you can use Musicfy for text-to-music generation and Magenta for additional manipulation, like MIDI-to-audio conversion. You can also leverage cloud storage to store generated files on platforms like AWS S3 for easy access in distributed applications. Finally, using Python to build real-time applications where users can create and play music interactively is possible.
Unlocking Musicfy: Your Key to AI Music Creation
Musicfy is an AI music generator that enables you to create your voice clone. You can create AI music with AI voices so that your song is free from being copyrighted with zero royalties. Musicfy uses a custom AI model that combines two different voices to create a unique voice that no other human has. This protects Musicfy users from copyright laws. The tool’s most prominent feature is text-to-music.
It enables you to describe a style of music and instrumentals and use AI to create the entire song in seconds—from voice to beat to everything that makes up a song! Musicfy also has a flagship feature that allows you to create the sound of an instrument with your voice. With voice-to-instrument, you can create the sound of a guitar and get the exact guitar sound created for you in seconds! Start using Musicfy’s AI voice generator for free today!
Create Viral Music In Seconds For Free with Musicfy's AI Music Generator
Musicfy is an AI music generator that has taken the music world by storm. The tool enables you to create your voice clone. This means you can make AI music with AI voices so that your song is free from being copyrighted – with zero royalties. Musicfy uses a custom AI model that combines two different voices to create a unique voice that no other human has. This protects Musicfy users from copyright laws. If you want to make music without the hassle of legal issues, Musicfy is an excellent option.
Create Unique Music in Seconds with Text-to-Music
Musicfy’s flagship feature is text-to-music. This groundbreaking AI music generator feature enables you to describe a style of music and instrumentals and use AI to create it the entire song in seconds – from voice to beat to everything that makes up a song!
Voice to Instrument: Create Unique Sounds with Musicfy
Musicfy also has a flagship feature that allows you to create the sound of an instrument with your voice (instrument voice – generate the sound of a guitar and get the exact guitar sound created for you in seconds)! Use Musicfy’s AI voice generator for free today! Create Viral Music In Seconds For Free with Musicfy's AI Music Generator.
Related Reading
• Soundraw Alternative
• Mubert Alternatives
• Suno AI Alternative
• Musicgen Alternative
• Splash Pro Alternative